What do Reddit, Instagram and Spotify have in common? Substantial parts of their backends are written in Python! Nevertheless, it is still widely believed that Python is just a scripting language that is not suitable for “real” software development. Let’s dispel this myth. We’ll discover that many objections are outdated because the Python ecosystem has evolved in the meantime. If you have the right techniques at hand to summon the wild Python snake, it becomes quite tame!
Lacking Types? Turn Dynamic Into Static!
One of the biggest objections to Python is that the language is dynamically typed. When a string method is called on a specific value, the interpreter has no way of checking the validity of this operation in advance. It simply tries, and if it succeeds, then it was probably a string. This is duck typing: If it looks like a duck, walks like a duck and quacks like a duck, then it just may be a duck.
Is this the death blow for larger software projects? Don’t worry, it’s not all doom and gloom yet: Nowadays, it is established practice to add type annotations to Python code. This unlocks superpowers in the IDE (keyword: type hints), and in automated tests, tools such as mypy can check the code for type safety. If this is not enough, you can even use typeguard to enforce type safety at runtime.
Activate the Turbo Gear
“Too slow” is the common verdict. In certain contexts, this is true: CPU-intensive tasks in neuralgic areas should not be executed in Python. Nevertheless, you can implement them in Python with ease! Pardon? Yes, indeed. The following options are available to speed up Python:
- The easiest way is not to use the standard CPython interpreter, but pypy. This way you can achieve a considerable performance gain without any additional effort. Unfortunately, this only applies under certain circumstances.
- But it is always possible to transpile your entire codebase (or parts of it) to C using mypyc, which makes it significantly faster. This only works with typed code – but you add type annotations to your code anyway, don’t you?
- The latest and most exciting raising star is Mojo, a superset of Python. By leveraging innovative compiler technologies, it plays (performance-wise) in the same league than Rust. Once Mojo is ready for production, many possibilities will open up here.
Thanks to Pydantic, We Can Be Pedantic
Sometimes, Python has nasty surprises in store. One of Python’s greatest strengths is also its greatest weakness: everything can be modified. At no point do we have the guarantee that an object has not been changed at runtime. Private fields only exist by convention, they can be changed from anywhere. With setattr() you can even attach completely arbitrary attributes to any object.
Fortunately, some pedantic members of the Python community came up with a solution: pydantic. Simply define validators for those classes where you need the objects to fulfill certain conditions. Pydantic then enforces these validation rules at runtime.
Rest In Peace, requirements.txt – Thanks to UV, Our Builds Are Now Reproducible
One thing that bothered me early on in my Python career was the chaotic way in which dependencies are managed. By default, dependencies are always installed globally (shudder!). It takes extra effort to create isolated project environments. But even then, there is no way to distinguish between deliberately installed dependencies vs. transitive ones (i.e. the dependencies of the dependencies). This quickly results in a huge number of dependencies, where nobody can tell what is really needed and what could be uninstalled.
It is also not possible to control the versions of the transitive dependencies. This can lead to a situation where the automated test suite runs successfully, but a user installation 5 minutes later leads to an error.
The fact that the interpreter version cannot be specified is also not exactly conducive to obtaining reproducible builds.
By the way – how many Python versions do you have installed, scattered all over your hard disk? Because every installer puts them at another place?
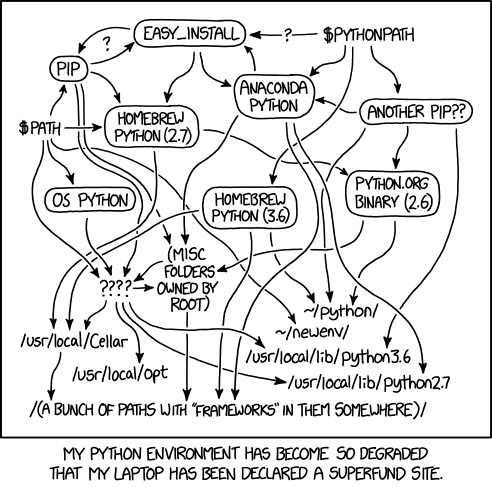
Fortunately, the community has stepped into the breach and developed UV, an advanced package manager and Python bootstrapping tool. UV not only solves all the problems mentioned above, but also truly sets new standards in terms of developer experience. It has gained a lot of momentum recently, slowly becoming the de facto standard. You should definitely give it a try!
Are Ruff And UV About to Unify the Scattered Ecosystem?
What did you just grumble to yourself? “Please spare me the 1000th Python package. I’m fed up with this fragmented ecosystem where everyone uses a different tool!” Unfortunately, there is indeed a multitude of formatters, linters, Python installers and environment managers. However, in recent years, not only has UV gained amazing momentum, but also Ruff. This formatter and linter reimplements the functionality of dozens of old-fashioned and slow tools, unified under a single roof. Thanks to Rust, it is orders of magnitude faster – a truly fantastic developer experience!
There is realistic hope that UV and Ruff will become a de facto standard. Can we soon say goodbye to the scattered ecosystem?
Well, Should I Then Choose Python for My Next Project?
This depends on your use case and your personal preference. If you and your team are experienced and knowledgeable in language X, it’s probably best to stick with it. But if you need to switch anyway, then Python is an interesting candidate:
- Python is beginner friendly. You’ll be productive quicker than in almost any other language.
- Therefore, recruiting is easier, because the developer pool is massive.
- No matter what problem you’re trying to solve, there’s a Python package for it. Seriously. Python is the second-best solution for virtually every problem you’ll ever encounter.
- Python is ideal for prototyping and for fast iteration. You may begin in “dirty mode” to check things out, and then later make your code more robust. This is interesting especially for startups.
- If you miss a feature in the core language, or if you’re unhappy with the syntax, you’re able to change the behavior of Python. Python has powerful metaprogramming features. Everything can be modified at any time. There are many popular Python packages that enhance developer experience by tweaking the standard behavior of the core language.
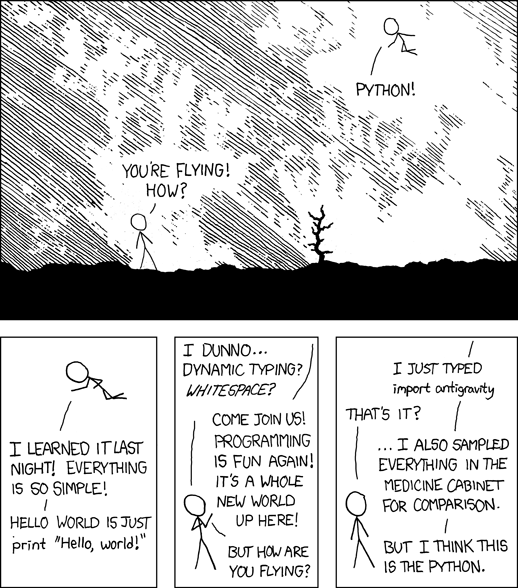